In-App Purchase
Architecture
This guide assumes that you have already Google Play billing integrated in your app. It will help you migrating from the Google Play to Catappult Billing service. Catappult billing service is implemented in a Wallet called "AppCoins Wallet". AppCoins wallet is widely available in different app stores. AppCoins Wallet exposes a Android Service which your app should bind with. Once bound to AppCoinsWallet service, your app can start communicating over IPC using an AIDL interface.
Google Play IAB to AppCoins IAB Migration Guide
Requirements
- AppCoins Public Key (retrieved from Catappult)
- Wallet Support
- Integration
- AIDL
- Permissions
- Service Connection
- Purchase Broadcast
Requirements
Catappult Public Key
Just like Google Play IAB, AppCoins IAB also exposes a public key. You should use AppCoins IAB public key to verify your purchases. It works exactly like Google Play IAB key, so you just need to replace one for the other.To find your Catappult public key, you should be registered in Catappult Back Office. You should head to Add App section of the BO and add the package name of your app (e.g. com.appcoins.wallet). Please make sure it's the same package name of the APK you'll upload later. Once you insert the package name and click "Next", you should already see your public key.
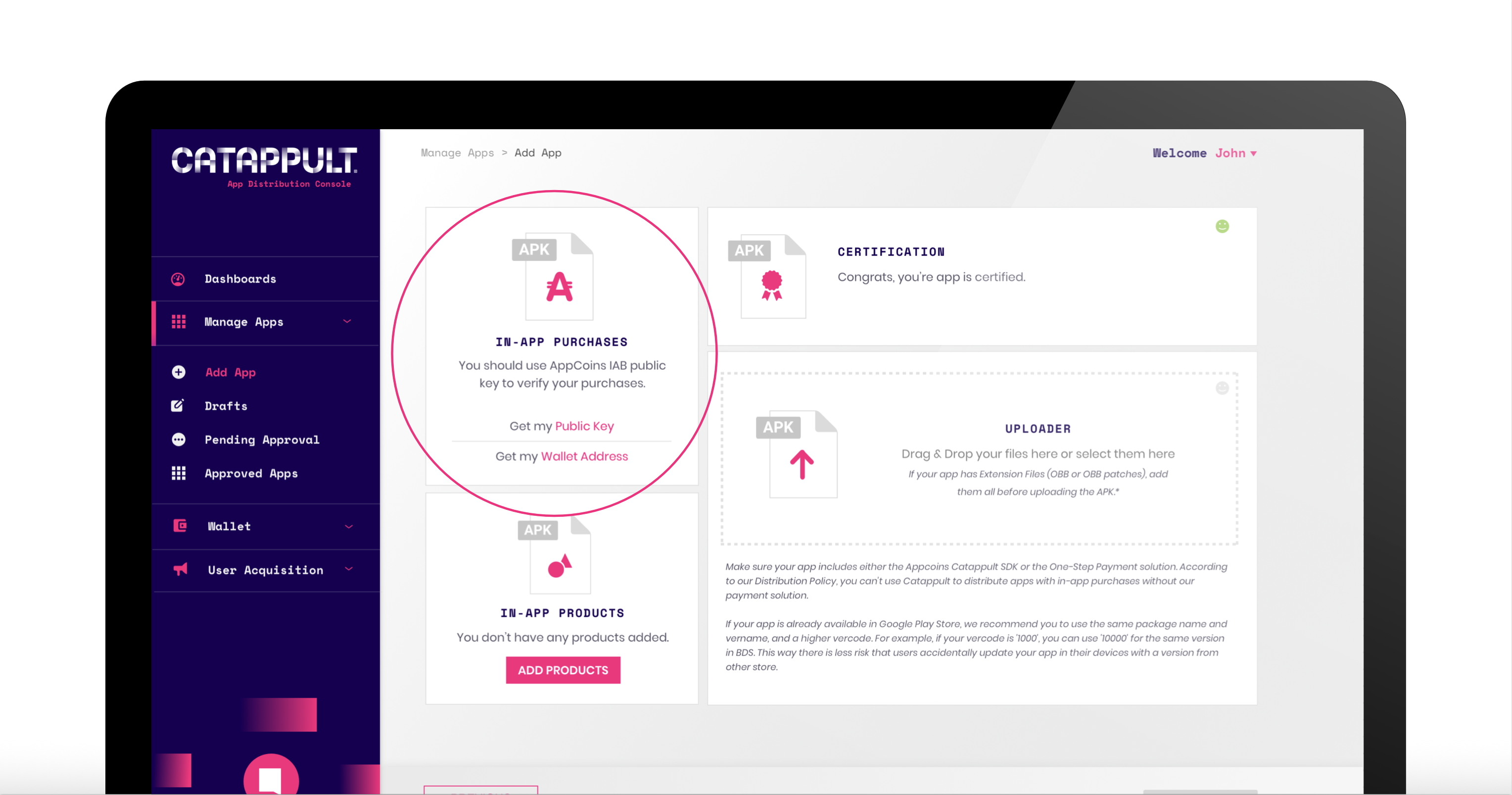
And change in your code:
String base64EncodedPublicKey = "MIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAzZZoGzIM+TlYDyGWl78g37ChRtrUWCOTnF8Sy4zwC2vqQhI/QETkYM/jMruY3pLvdDOcdWm9xoZLJq5exxqQyhrZbSoziXQ9bIOJwNSjA/WIJnAewAk8QGPhvjNFy0wflCqLDWflrt04vbvvNsdh9UpgceIdinzqE0MhF94HJr1Ovb5JEMktDgU89SarCsx90a4Psk6cdapulGjdpL35dBRkRkM74sfNmSy8Oan9FaI36+z4h88MgTrELHnQ0XTlS32flvCK7nhICV+bzcY89wPRPN4rkqjS4F5nngwAnaz5VMV0Zy5SZBZntLS1QIA641FxJJHAikM8ZYz3hkTn0QIDAQAB";
Wallet Support
In order to use AppCoins, you will need a compliant wallet installed. The following snippets shows how you can prompt the user to install the AppCoins Wallet when not present.We recommend you to do this validation when the user intends to do a purchase. By doing so, you avoid misleading the user on stating a purchase without the wallet installed. To do that, first validate if the Appcoins Wallet is installed.
if (!hasWalletInstalled(this)) {
showWalletInstallDialog(this,
this.getString(R.string.install_wallet_from_iab));
}
To check if the AppCoins Wallet is installed, try to obtain the information for the wallet package name. It can be done with the following.
public static boolean hasWalletInstalled(Context context) {
PackageManager packageManager = context.getPackageManager();
try {
packageManager.getPackageInfo("com.appcoins.wallet", 0);
return true;
} catch (PackageManager.NameNotFoundException e) {
return false;
}
}
Showing a basic dialog that informs the user of the need to install the AppCoins wallet can be done with the following method. Notice that the dialog generated by this method is basic and provides the minimum required information. The creation of a new dialog is advised, to be compatible with the layout of your application.
private static void showWalletInstallDialog(Context context, String message) {
AlertDialog.Builder builder;
builder = new AlertDialog.Builder(context);
builder.setTitle(R.string.wallet_missing)
.setMessage(message)
.setPositiveButton(R.string.install, (dialog, which) -> gotoStore(context))
.setNegativeButton(R.string.skip, (DialogInterface dialog, int which) -> dialog.dismiss())
.setIcon(android.R.drawable.ic_dialog_alert)
.show();
}
If you follow with the use of the code above the resources below are required.
<string name="install">INSTALL</string>
<string name="skip">SKIP</string>
<string name="install_wallet_from_iab">To pay for in-app items, you need to install the AppCoins Wallet.</string>
<string name="wallet_missing">AppCoins Wallet Missing</string>
The following method is the one used to redirect the user to AppCoins Wallet store page.
@NonNull private static void gotoStore(Context activity) {
String appPackageName = "com.appcoins.wallet";
try {
activity.startActivity(
new Intent(Intent.ACTION_VIEW, Uri.parse("market://details?id=" + appPackageName)));
} catch (android.content.ActivityNotFoundException anfe) {
activity.startActivity(new Intent(Intent.ACTION_VIEW,
Uri.parse("https://play.google.com/store/apps/details?id=" + appPackageName)));
}
}
Integration
1. AIDL
Like Google Play IAB, AppCoins IAB uses an AIDL file in order to communicate with AppCoins service. The AppCoins IAB file is here.
In Android Studio, follow the steps:
- rename InAppBillingService.aidl (Google AIDL) file to AppcoinsBilling.aidl: in Android Studio, select the file InAppBillingService.aidl, right click and choose the option Refactor -> Rename.) and change it to AppcoinsBilling.aidl.
- Open AppcoinsBilling.aidl (InAppBillingService.aidl ), erase all the lines and replace by the content of AppCoins AIDL file.
- the path to the AppcoinsBilling.aidl file needs to follow the package name structure (e.g. aidl/com/appcoins/billing). In the root of yourAndroid studio project, move the file to a new directory:
$ find . -name "*aidl"
./app/src/main/aidl/com/android/vending/billing/AppcoinsBilling.aidl
$ mkdir -p ./app/src/main/aidl/com/appcoins/billing/
$ mv ./app/src/main/aidl/com/android/vending/billing/AppcoinsBilling.aidl ./app/src/main/aidl/com/appcoins/billing/
- Change all occurrences of "IInAppBillingService" to "AppcoinsBilling" and also all occurrences of "com.android.vending" to "com.appcoins.billing". Right click on the root of your Java files and choose "Replace in path" option works.
There are some methods of Google Billing (like getBuyIntentToReplaceSkus) that may not be supported yet. In those cases, change the code to use the existent ones.
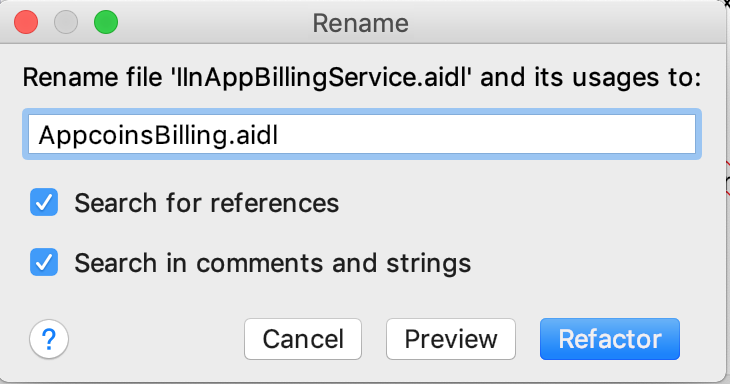
2. Permissions
Your app needs a permission to allow it to perform billing actions with AppCoins IAB. The permission is declared in the AndroidManifest.xml file of your app. Since Google Play IAB already declares a permission with name com.android.vending.BILLING, you should rename it to com.appcoins.BILLING.### Google Play IAB
<uses-permission android:name="com.android.vending.BILLING" />
AppCoins IAB
<uses-permission android:name="com.appcoins.BILLING" />
3. Service Connection
In order to communicate with AppCoins IAB, your app must bind to a service in the same way as in Google Play IAB. Google Play IAB Intent action and package must be updated from com.android.vending.billing.InAppBillingService.BIND and com.android.vending to com.appcoins.wallet.iab.action.BIND and com.appcoins.wallet, respectively.
Intent serviceIntent = new Intent("com.android.vending.billing.InAppBillingService.BIND");
serviceIntent.setPackage("com.android.vending");
AppCoins IAB Service Intent
Intent serviceIntent = new Intent("com.appcoins.wallet.iab.action.BIND");
serviceIntent.setPackage("com.appcoins.wallet");
You can find & replace in the Android Studio for the above strings. Depending on the approach taken for Google Billing integration, it can be in different files (e.g. IabHelper.java).
Payment Intent
When calling getPaymentIntent method, you must send your developer's Ethereum wallet address. This can be done by using the helper provided here.
...
String payload = PayloadHelper.buildIntentPayload("developer_ethereum_address","developer_payload");
Bundle buyIntentBundle = mService.getBuyIntent(3, mContext.getPackageName(), sku, itemType, payload);
In order to get your developer's Ethereum wallet address, go to Catappult Back Office -> Wallet -> Deposit APPC and click on "copy to clipboard button".## 4. Purchase Broadcast
Google Play IAB broadcasts and Intent with action com.android.vending.billing.PURCHASES_UPDATED. Since AppCoins IAB does not implement this, any code related with listening to that Intent can be removed.
Testing
For testing purposes you can use the following data to test the billing of you application.* applicationId: com.appcoins.sample
- IAB_KEY: MIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAyEt94j9rt0UvpkZ2jPMZZ16yUrBOtjpIQCWi/F3HN0+iwSAeEJyDw7xIKfNTEc0msm+m6ud1kJpLK3oCsK61syZ8bYQlNZkUxTaWNof1nMnbw3Xu5nuYMuowmzDqNMWg5jNooy6oxwIgVcdvbyGi5RIlxqbo2vSAwpbAAZE2HbUrysKhLME7IOrdRR8MQbSbKEy/9MtfKz0uZCJGi9h+dQb0b69H7Yo+/BN/ayBSJzOPlaqmiHK5lZsnZhK+ixpB883fr+PgSczU7qGoktqoe6Fs+nhk9bLElljCs5ZIl9/NmOSteipkbplhqLY7KwapDmhrtBgrTetmnW9PU/eCWQIDAQAB
Known Issues
- AppCoins IAB is not compliant with Google Play IAB v5. Calls to getBuyIntentToReplaceSkus method will always fail.
Updated over 4 years ago